An array is collection of items stored at continuous memory locations. The idea is to declare multiple items of same type together.
Overview:
- An array is a collection of data items, all of the same type, accessed using a common name.
- A one-dimensional array is like a list; A two dimensional array is like a table; The C language places no limits on the number of dimensions in an array, though specific implementations may.
- Some texts refer to one-dimensional arrays as vectors, two-dimensional arrays as matrices, and use the general term arrays when the number of dimensions is unspecified or unimportant.
An Example to show that array elements are stored at contiguous locations
// C program to demonstrate that array elements are stored
// contiguous locations
int main()
{
// an array of 10 integers. If arr[0] is stored at
// address x, then arr[1] is stored at x + sizeof(int)
// arr[2] is stored at x + sizeof(int) + sizeof(int)
// and so on.
int arr[5], i;
printf("Size of integer in this compiler is %u\n", sizeof(int));
for (i=0; i<5; i++)
// The use of '&' before a variable name, yields
// address of variable.
printf("Address arr[%d] is %u\n", i, &arr[i]);
return 0;
}
Instead of declaring individual variables, such as number0, number1, ..., and number99, you declare one array variable such as numbers and use numbers[0], numbers[1], and ..., numbers[99] to represent individual variables. A specific element in an array is accessed by an index.
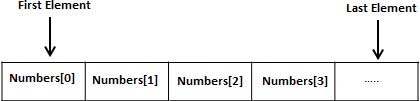
Declaring Arrays
- Array variables are declared identically to variables of their data type, except that the variable name is followed by one pair of square [ ] brackets for each dimension of the array.
- Uninitialized arrays must have the dimensions of their rows, columns, etc. listed within the square brackets.
- Dimensions used when declaring arrays in C must be positive integral constants or constant expressions.
- Examples:
int i, j, intArray[ 10 ], number; float floatArray[ 1000 ]; int tableArray[ 3 ][ 5 ]; /* 3 rows by 5 columns */ const int NROWS = 100; // ( Old code would use #define NROWS 100 ) const int NCOLS = 200; // ( Old code would use #define NCOLS 200 ) float matrix[ NROWS ][ NCOLS ];
// Array declaration by specifying size int arr[10];
arr[] = {10, 20, 30, 40} |
// Compiler creates an array of size 4.
// above is same as "int arr[4] = {10, 20, 30, 40}"
// Array declaration by specifying size and initializing
// elements
int
arr[6] = {10, 20, 30, 40}
// Compiler creates an array of size 6, initializes first
// 4 elements as specified by user and rest two elements as 0.
// above is same as "int arr[] = {10, 20, 30, 40, 0, 0}"
Accessing Array Elements in C:
let us learn this topic with an example:
int
main()
{
int
arr[5];
arr[0] = 5;
arr[2] = -10;
arr[3/2] = 2;
// this is same as arr[1] = 2
arr[3] = arr[0];
printf
(
"%d %d %d %d"
, arr[0], arr[1], arr[2], arr[3]);
return
0;
}
⇒C Compiler to run above code:http://bit.ly/2uPGImG
EXAMPLE FOR C ARRAYS:
int a[10]; // integer array
char b[10]; // character array i.e. string
char b[10]; // character array i.e. string
TYPES OF ARRAYS IN C:
1.One dimensional array2.Multi dimensional array
- Two dimensional array
- Three dimensional array
- four dimensional array etc…
1 D Array:
Array declaration, initialization and accessing
|
Example
|
Array declaration syntax:
data_type arr_name [arr_size];
Array initialization syntax:
data_type arr_name [arr_size]=(value1, value2, value3,….);
Array accessing syntax:
arr_name[index]; | Integer array example:
int age [5];
int age[5]={0, 1, 2, 3, 4};
age[0]; /*0 is accessed*/
age[1]; /*1 is accessed*/ age[2]; /*2 is accessed*/ |
Character array example:
char str[10];
char str[10]={‘H’,‘a’,‘i’}; (or) char str[0] = ‘H’; char str[1] = ‘a’; char str[2] = ‘i;
str[0]; /*H is accessed*/
str[1]; /*a is accessed*/ str[2]; /*i is accessed*/ |
Sample Programs Using 1-D Arrays:
/* Program to calculate the first 20 Fibonacci numbers. */
#include <stdlib.h>
#include <stdio.h>
int main( void ) {
int i, fibonacci[ 20 ];
fibonacci[ 0 ] = 0;
fibonacci[ 1 ] = 1;
for( i = 2; i < 20; i++ )
fibonacci[ i ] = fibonacci[ i - 2 ] + fibonacci[ i - 1 ];
for( i = 0; i < 20; i++ )
printf( "Fibonacci[ %d ] = %f\n", i, fibonacci[ i ] );
} /* End of sample program to calculate Fibonacci numbers */
2D Array:
Array declaration, initialization and accessing
|
Example
|
Array declaration syntax: data_type arr_name [num_of_rows][num_of_column];
Array initialization syntax:
data_type arr_name[2][2] = {{0,0},{0,1},{1,0},{1,1}};
Array accessing syntax:
arr_name[index]; | Integer array example:
int arr[2][2];
int arr[2][2] = {1,2, 3, 4};
arr [0] [0] = 1;arr [0] ]1] = 2;arr [1][0] = 3;arr [1] [1] = 4;
|
2D array is nothing but Array of an Array
Example for 2D array:
#include <stdlib.h>
#include <stdio.h>
int main( void ) {
/* Program to add two multidimensional arrays */
/* Written May 1995 by George P. Burdell */
int a[ 2 ][ 3 ] = { { 5, 6, 7 }, { 10, 20, 30 } };
int b[ 2 ][ 3 ] = { { 1, 2, 3 }, { 3, 2, 1 } };
int sum[ 2 ][ 3 ], row, column;
/* First the addition */
for( row = 0; row < 2; row++ )
for( column = 0; column < 3; column++ )
sum[ row ][ column ] =
a[ row ][ column ] + b[ row ][ column ];
/* Then print the results */
printf( "The sum is: \n\n" );
for( row = 0; row < 2; row++ ) {
for( column = 0; column < 3; column++ )
printf( "\t%d", sum[ row ][ column ] );
printf( '\n' ); /* at end of each row */
}
return 0;
}
No comments:
Post a Comment