A pointer is a variable that stores the address of another variable. Unlike other variables that hold values of a certain type, pointer holds the address of a variable.
For example, an integer variable holds (or you can say stores) an integer value, however an integer pointer holds the address of a integer variable.
Every variable is a memory location and every memory location has its address defined which can be accessed using ampersand (&) operator, which denotes an address in memory.
It is always a good practice to assign a NULL value to a pointer variable in case you do not have an exact address to be assigned. This is done at the time of variable declaration. A pointer that is assigned NULL is called a nullpointer.
The NULL pointer is a constant with a value of zero defined in several standard libraries. Consider the following program −
#include <stdio.h>
int main () {
int *ptr = NULL;
printf("The value of ptr is : %x\n", ptr );
return 0;
}
When the above code is compiled and executed, it produces the following result −
The value of ptr is 0
In most of the operating systems, programs are not permitted to access memory at address 0 because that memory is reserved by the operating system. However, the memory address 0 has special significance; it signals that the pointer is not intended to point to an accessible memory location. But by convention, if a pointer contains the null (zero) value, it is assumed to point to nothing.
To check for a null pointer, you can use an 'if' statement as follows −
if(ptr) /* succeeds if p is not null */
if(!ptr) /* succeeds if p is null */
Dereferencing:
/* define a local variable a */
int a = 1;
/* define a pointer variable, and point it to a using the & operator */
int * pointer_to_a = &a;
printf("The value a is %d\n", a);
printf("The value of a is also %d\n", *pointer_to_a);
For example, an integer variable holds (or you can say stores) an integer value, however an integer pointer holds the address of a integer variable.
Every variable is a memory location and every memory location has its address defined which can be accessed using ampersand (&) operator, which denotes an address in memory.
Pointer Declaration :
Syntax:(type) *var-name;
Example:
int *ip; /* pointer to an integer */
int *ip; /* pointer to an integer */
double *dp; /* pointer to a double */
float *fp; /* pointer to a float */
Pointer Use Using Code:
#include <stdio.h>
int main()
{
int x = 10;
int* px = &x;
printf("Direct access, x = %d\n",x);
printf("Indirect access, *px = %d\n",*px);
*px = 20;
printf("Direct access, x = %d\n",x);
printf("Indirect access, *px = %d\n",*px);
/* examining memory address of x and px */
printf("The memory address of x is %p\n",&x);
printf("The value of the pointer px is %p\n",px);
printf("The memory address of the pointer px is %p\n",&px);
return 0;
}
C output:
Direct access, x = 10
Indirect access, *px = 10
Direct access, x = 20
Indirect access, *px = 20
The memory address of x is 0x7fffc97820cc
The value of the pointer px is 0x7fffc97820cc
The memory address of the pointer px is 0x7fffc97820d0
Direct access, x = 10
Indirect access, *px = 10
Direct access, x = 20
Indirect access, *px = 20
The memory address of x is 0x7fffc97820cc
The value of the pointer px is 0x7fffc97820cc
The memory address of the pointer px is 0x7fffc97820d0
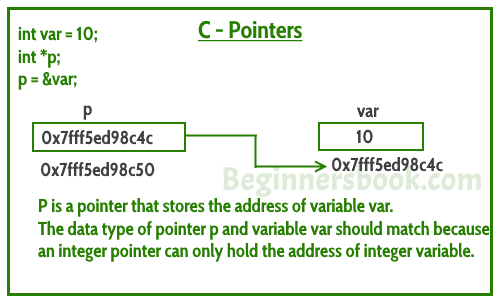
C Compiler to run above code:https://goo.gl/bzuHcQ
NULL Pointers :
It is always a good practice to assign a NULL value to a pointer variable in case you do not have an exact address to be assigned. This is done at the time of variable declaration. A pointer that is assigned NULL is called a nullpointer.
The NULL pointer is a constant with a value of zero defined in several standard libraries. Consider the following program −
#include <stdio.h>
int main () {
int *ptr = NULL;
printf("The value of ptr is : %x\n", ptr );
return 0;
}
When the above code is compiled and executed, it produces the following result −
The value of ptr is 0
In most of the operating systems, programs are not permitted to access memory at address 0 because that memory is reserved by the operating system. However, the memory address 0 has special significance; it signals that the pointer is not intended to point to an accessible memory location. But by convention, if a pointer contains the null (zero) value, it is assumed to point to nothing.
To check for a null pointer, you can use an 'if' statement as follows −
if(ptr) /* succeeds if p is not null */
if(!ptr) /* succeeds if p is null */
Dereferencing:
Dereferencing is the act of referring to where the pointer points at, instead of the memory address. We are already using dereferencing in arrays - but we just didn't know it yet. The brackets operator -
[0]
for example, accesses the first item of the array. And since arrays are actually pointers, accessing the first item in the array is the same as dereferencing a pointer. Dereferencing a pointer is done using the asterisk operator *
/* define a local variable a */
int a = 1;
/* define a pointer variable, and point it to a using the & operator */
int * pointer_to_a = &a;
printf("The value a is %d\n", a);
printf("The value of a is also %d\n", *pointer_to_a);
No comments:
Post a Comment